ISS Fly Over notification API
We are building today a Python API that uses the Open Notify API to get the International Space Station (ISS) position and sends a notification to the user when the ISS is right above Geneva, Switzerland.
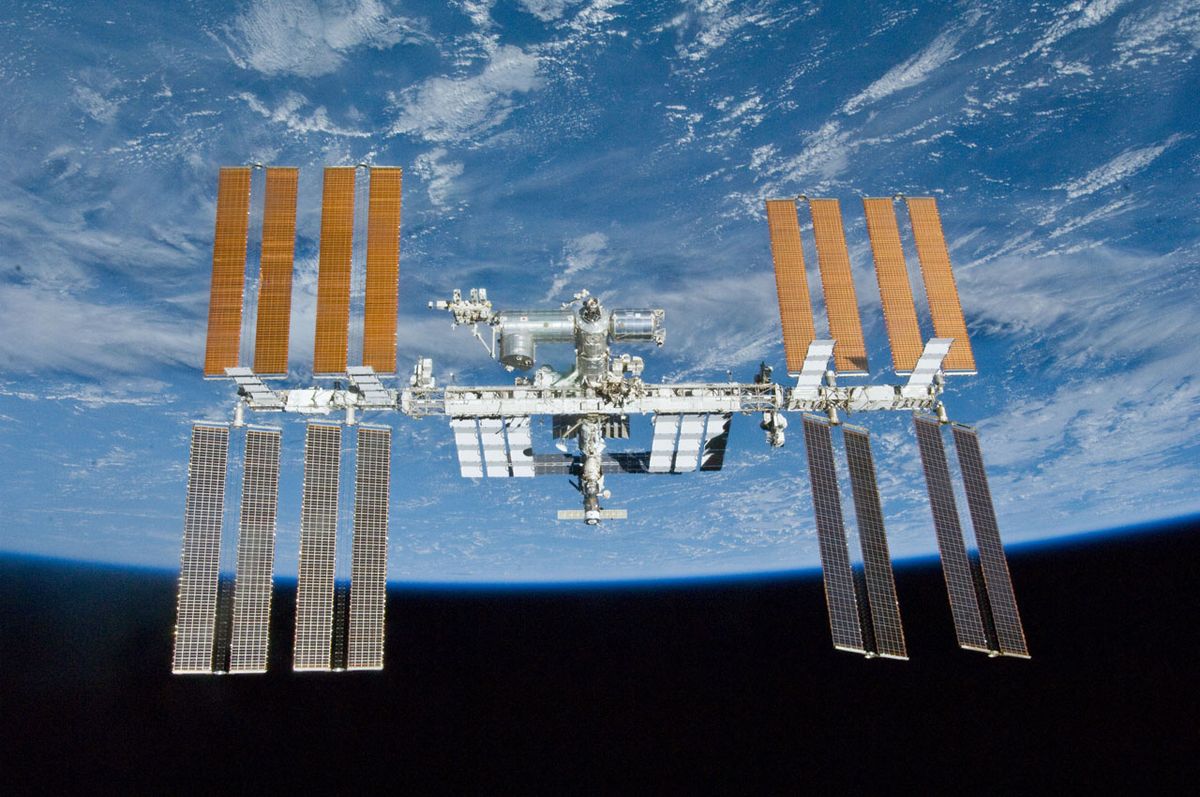
We are building today a Python API that uses the Open Notify API to get the International Space Station (ISS) position and sends a notification to the user when the ISS is right above Geneva, Switzerland.
Python Code
First, make sure you have Docker installed on your machine. Then, follow these steps:
Create a new directory for your project and navigate into it:
mkdir iss-notifier cd iss-notifier
Create a new Python file called app.py
and open it in a text editor. Add the following code:
import requests
import time
from plyer import notification
def get_iss_position():
response = requests.get("http://api.open-notify.org/iss-now.json")
data = response.json()
return float(data["iss_position"]["latitude"]), float(data["iss_position"]["longitude"])
def notify_user():
while True:
latitude, longitude = get_iss_position()
if 46.2044 <= latitude <= 46.3050 and 6.0847 <= longitude <= 6.1548:
notification.notify(
title="ISS Notification",
message="The ISS is right above Geneva, Switzerland!",
timeout=10
)
time.sleep(60) # Check every minute
if __name__ == "__main__":
notify_user()
This code defines two functions: get_iss_position()
retrieves the current ISS position using the Open Notify API, and notify_user()
checks if the ISS is above Geneva and sends a notification using the Plyer library.
Create a new file called requirements.txt
and add the following content:
requests plyer
This file specifies the Python dependencies required for our application.
Create a new file called Dockerfile
(without any file extension) and add the following content:
FROM python:3.9-slim-buster WORKDIR /app COPY requirements.txt . RUN pip install --no-cache-dir -r requirements.txt COPY . . CMD [ "python", "./app.py" ]
This Dockerfile sets up a Docker image based on the official Python 3.9 slim-buster image, installs the required dependencies, and runs our app.py
script.
Open a terminal, navigate to the project directory (iss-notifier
), and build the Docker image:
docker build -t iss-notifier .
Once the image is built, run a Docker container from the image:
docker run -d --name iss-notifier-container iss-notifier
This will start the container in the background, and the ISS notifier will run continuously within the container.
Now, whenever the ISS is right above Geneva, Switzerland, a notification will be sent to your system. You can customize the notification properties (e.g., sound, timeout) in the notify_user()
function.
Note: Make sure you have a desktop environment that supports system notifications, and also ensure that your Docker container has access to the internet to make API requests to the Open Notify API.
Unit tests for the ISS Notifier
import unittest
from unittest.mock import MagicMock, patch
import requests
from plyer import notification
from iss_tracker import get_iss_position, notify_user
class TestISSPosition(unittest.TestCase):
def test_get_iss_position(self):
# Mock the response from the API
mock_response = MagicMock()
mock_response.json.return_value = {
"iss_position": {
"latitude": "46.1234",
"longitude": "6.5678"
}
}
with patch("requests.get", return_value=mock_response):
latitude, longitude = get_iss_position()
self.assertEqual(latitude, 46.1234)
self.assertEqual(longitude, 6.5678)
def test_notify_user_in_geneva(self):
mock_get_iss_position = MagicMock(return_value=(46.2500, 6.1200))
with patch("iss_tracker.get_iss_position", mock_get_iss_position):
with patch.object(notification, "notify") as mock_notify:
notify_user()
mock_notify.assert_called_once_with(
title="ISS Notification",
message="The ISS is right above Geneva, Switzerland!",
timeout=10
)
def test_notify_user_not_in_geneva(self):
mock_get_iss_position = MagicMock(return_value=(50.0000, 10.0000))
with patch("iss_tracker.get_iss_position", mock_get_iss_position):
with patch.object(notification, "notify") as mock_notify:
notify_user()
mock_notify.assert_not_called()
if __name__ == "__main__":
unittest.main()
These tests cover the get_iss_position
function and the notify_user
function, checking if the notification is correctly triggered when the ISS is above Geneva and if it is not triggered when the ISS is not above Geneva. The requests.get
function is mocked to return a predefined JSON response for testing purposes.