Introduction to Machine Learning with Python: A Beginner's Guide
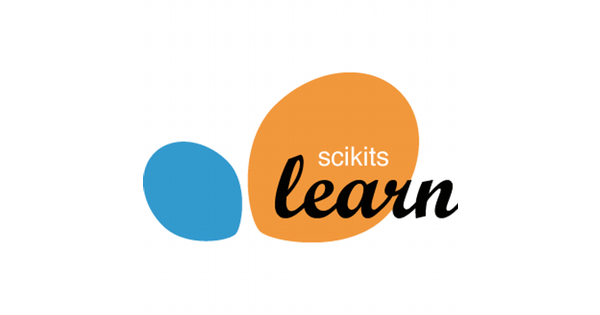
Machine learning is a rapidly growing field that involves developing algorithms and models that enable computers to learn and make predictions or decisions without being explicitly programmed. Python, with its simplicity and extensive libraries, has become one of the go-to programming languages for machine learning tasks. In this beginner's guide, we will explore the basics of machine learning with Python and provide practical examples along with Python code snippets.
What is Machine Learning?
Machine learning is a subset of artificial intelligence that focuses on the development of algorithms that can learn from and make predictions or decisions based on data. It involves training a model on a dataset and using the trained model to make predictions or decisions on new, unseen data.
Python Libraries for Machine Learning
Python provides several powerful libraries for machine learning. Some of the most popular ones are:
NumPy: NumPy is a fundamental library for scientific computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
Pandas: Pandas is a library that provides easy-to-use data structures and data analysis tools. It is built on top of NumPy and is particularly useful for handling structured data, such as CSV files or SQL tables.
Scikit-learn: Scikit-learn is a machine learning library that provides a wide range of algorithms and tools for classification, regression, clustering and dimensionality reduction. It is built on top of NumPy, SciPy and Matplotlib.
TensorFlow: TensorFlow is an open-source machine learning framework developed by Google. It provides a flexible and efficient way to build and deploy machine learning models across different platforms.
Steps in a Machine Learning Project
A typical machine learning project involves the following steps:
Data Collection: The first step is to collect the relevant data for your machine learning task. This can involve collecting data from various sources, such as databases, APIs, or web scraping.
Data Preprocessing: Once you have collected the data, you need to preprocess it to make it suitable for training a machine learning model. This step may involve handling missing values, dealing with outliers and scaling or normalising the data.
Feature Engineering: Feature engineering involves selecting or creating relevant features from the available data. This step can significantly impact the performance of your machine learning model.
Model Selection: The next step is to select an appropriate machine learning algorithm for your task. This decision depends on the type of problem you are trying to solve (e.g., classification, regression, or clustering) and the nature of your data.
Model Training: Once you have selected a model, you need to train it on your training dataset. This involves feeding the model with the input features and the corresponding target values and adjusting its internal parameters to minimise the prediction error.
Model Evaluation: After training the model, you need to evaluate its performance on a separate validation dataset. This step helps you assess how well your model generalises to unseen data.
Model Deployment: Once you are satisfied with the performance of your model, you can deploy it to make predictions or decisions on new, unseen data.
Example: Classifying Iris Flowers using Scikit-learn
Let's walk through a simple example of using Scikit-learn to classify iris flowers. The iris dataset is a popular dataset in machine learning, which contains measurements of various iris flowers along with their corresponding species.
First, let's import the necessary libraries and load the iris dataset:
import numpy as np
from sklearn import datasets
# Load the iris dataset
iris = datasets.load_iris()
X = iris.data
y = iris.target
Next, let's split the dataset into training and testing sets:
from sklearn.model_selection import train_test_split
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Now, let's train a Support Vector Machine (SVM) classifier on the training data:
from sklearn.svm import SVC
# Create an SVM classifier
clf = SVC()
# Train the classifier on the training data
clf.fit(X_train, y_train)
Finally, let's evaluate the performance of the classifier on the testing data:
from sklearn.metrics import accuracy_score
# Make predictions on the testing data
y_pred = clf.predict(X_test)
# Calculate the accuracy of the classifier
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
This example demonstrates the basic steps involved in a machine learning project, from data loading and preprocessing to model training and evaluation.
Conclusion
In this beginner's guide, we introduced the basics of machine learning with Python. We explored the essential libraries for machine learning in Python, discussed the steps involved in a machine learning project and provided a practical example of classifying iris flowers using Scikit-learn. By following this guide and experimenting with different datasets and algorithms, you can start your journey into the exciting world of machine learning with Python.