Introduction to Ethereum: A Decentralized Smart Contract Platform
Ethereum is a decentralized, open-source blockchain platform that enables developers to build and deploy smart contracts. It was proposed by Vitalik Buterin in late 2013 and launched in 2015. Ethereum allows the creation of decentralized applications (dApps)
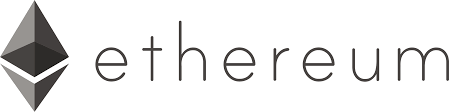
Overview
Ethereum is a decentralized, open-source blockchain platform that enables developers to build and deploy smart contracts. It was proposed by Vitalik Buterin in late 2013 and launched in 2015. Ethereum allows the creation of decentralized applications (dApps) that can run without any downtime, fraud, or interference from third parties.
Smart Contracts
Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They automatically execute when predefined conditions are met. Ethereum's smart contracts are written in a language called Solidity, which is similar to JavaScript.
Solidity Example
Here's an example of a simple smart contract written in Solidity:
pragma solidity ^0.8.0;
contract HelloWorld {
string public message;
constructor() {
message = "Hello, World!";
}
function setMessage(string memory newMessage) public {
message = newMessage;
}
}
In this example, we define a contract called HelloWorld
with a public variable message
. The constructor sets the initial value of message
to "Hello, World!". The setMessage
function allows anyone to update the value of message
.
Ethereum Virtual Machine (EVM)
Ethereum has its own virtual machine called the Ethereum Virtual Machine (EVM). It is a runtime environment that executes smart contracts on the Ethereum network. The EVM is responsible for executing the bytecode generated from the Solidity code.
Gas and Gas Fees
Gas is a measure of computational effort required to execute operations or run smart contracts on the Ethereum network. Each operation has a predefined gas cost associated with it. Gas fees are paid by users to compensate the network for the computational resources used.
Interacting with Ethereum
To interact with Ethereum, you can use various libraries and frameworks. One popular choice is the web3.js library, which provides a JavaScript API for interacting with Ethereum.
Web3.js Example
Here's an example of how to interact with the HelloWorld
smart contract using web3.js:
const Web3 = require('web3');
const contractAbi = require('./HelloWorld.abi.json');
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
const contractAddress = '0x1234567890abcdef1234567890abcdef12345678';
const contract = new web3.eth.Contract(contractAbi, contractAddress);
contract.methods.message().call((error, result) => {
if (error) {
console.error(error);
} else {
console.log(result);
}
});
contract.methods.setMessage('New message').send({ from: '0xYOUR_ADDRESS' })
.on('transactionHash', (hash) => {
console.log('Transaction hash:', hash);
})
.on('receipt', (receipt) => {
console.log('Transaction receipt:', receipt);
})
.on('error', (error) => {
console.error(error);
});
In this example, we create a web3 instance connected to the Ethereum mainnet using Infura as the provider. We then create a contract instance using the contract ABI (Application Binary Interface) and the contract address. We can call the message
function to retrieve the current message value and use the setMessage
function to update it.
Conclusion
Ethereum is a powerful platform for building decentralized applications and executing smart contracts. Its ability to execute code in a trustless and transparent manner opens up a wide range of possibilities for developers. With the example provided, you should now have a basic understanding of Ethereum and how to interact with it using Solidity and web3.js. Happy coding!