How to Use Streamlit in Python
Streamlit is an open-source Python library that allows you to create interactive web applications for machine learning and data science projects. It provides a simple and intuitive way to build beautiful and functional user interfaces
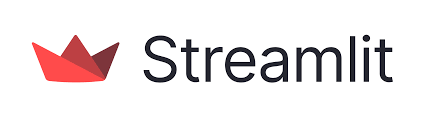
Introduction
Streamlit is an open-source Python library that allows you to create interactive web applications for machine learning and data science projects. It provides a simple and intuitive way to build beautiful and functional user interfaces without the need for web development skills. In this tutorial, we will explore the basics of using Streamlit and provide you with five coding examples to get you started.
Installation
To install Streamlit, you can use pip, the Python package installer. Open your terminal and run the following command:
pip install streamlit
Getting Started
Once Streamlit is installed, you can create a new Python file and import the library:
import streamlit as st
To run your Streamlit application, use the following command in your terminal:
streamlit run your_file.py
Now, let's dive into some coding examples to see what Streamlit can do!
Example 1: Hello World
import streamlit as st
st.title("Welcome to Streamlit!")
st.write("Hello, world!")
In this example, we import Streamlit and use the st.title
and st.write
functions to display a title and a simple message.
Example 2: Data Visualization
import streamlit as st
import pandas as pd
import matplotlib.pyplot as plt
data = pd.read_csv("data.csv")
st.title("Data Visualization")
st.line_chart(data)
In this example, we import Streamlit, Pandas, and Matplotlib to visualize data from a CSV file using a line chart.
Example 3: Interactive Widgets
import streamlit as st
name = st.text_input("Enter your name", "John Doe")
age = st.slider("Select your age", 0, 100, 25)
st.write(f"Hello, {name}! You are {age} years old.")
In this example, we use Streamlit's interactive widgets to get user input for name and age, and display a personalized message.
Example 4: Machine Learning Model
import streamlit as st
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
data = pd.read_csv("data.csv")
X = data.drop("target", axis=1)
y = data["target"]
model = RandomForestClassifier()
model.fit(X, y)
st.title("Machine Learning Model")
st.write("Enter some values:")
feature1 = st.slider("Feature 1", 0, 10, 5)
feature2 = st.slider("Feature 2", 0, 10, 5)
prediction = model.predict([[feature1, feature2]])
st.write(f"Prediction: {prediction}")
In this example, we import Streamlit, Pandas, and scikit-learn to build and deploy a simple machine learning model using a random forest classifier.
Example 5: Deployment
Once you have built your Streamlit application, you can deploy it using various platforms such as Heroku, AWS, or Homelab. Refer to the Streamlit documentation for detailed instructions on deployment.
Conclusion
Streamlit is a powerful tool for creating interactive web applications in Python. In this tutorial, we covered the basics of using Streamlit and provided five coding examples to help you get started. Experiment with these examples and explore the Streamlit documentation to unlock the full potential of this library.
Remember to install Streamlit using pip install streamlit
and run your Streamlit application with streamlit run your_file.py
.