GPT 3.5 Turbo Fine Tuning in Python
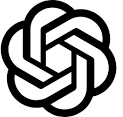
To fine-tune the GPT-3.5 model in Python, you can use OpenAI's tune
API. Here's an example code snippet to guide you through the process:
import openai
# Set OpenAI API key
openai.api_key = 'YOUR_API_KEY'
# Load your training data
with open('training_data.txt', 'r') as file:
training_data = file.read()
# Fine-tune the model
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": training_data}
],
training_configuration={
'language': 'en',
'use_case': 'fine_tuning',
'model_name': 'gpt-3.5-turbo'
}
)
# Retrieve the model ID
model_id = response['id']
# Wait for the fine-tuning process to complete
while True:
response = openai.ChatCompletion.retrieve(model_id)
if response['status'] == 'succeeded':
break
# Save the fine-tuned model
with open('fine_tuned_model.txt', 'w') as file:
file.write(response['model']['gpt3.5-turbo']['id'])
In this example, you first need to set your OpenAI API key using openai.api_key
. Then, you load your training data from a file (training_data.txt
) and pass it as the user message during fine-tuning. The messages
parameter is an array of message objects, where the system message sets the context for the conversation and the user message contains the training data.
Next, you use the openai.ChatCompletion.create
method to start the fine-tuning process. You specify the base model (gpt-3.5-turbo
), the messages, and the training configuration. The training_configuration
includes the language and the model name.
After making the API call, you retrieve the model ID from the response and wait for the fine-tuning process to complete. This is done by periodically calling openai.ChatCompletion.retrieve
until the status of the response is succeeded
.
Finally, you save the ID of the fine-tuned model to a file (fine_tuned_model.txt
) for future use.
Note: Fine-tuning the GPT-3 model is a complex process that requires careful handling of data and consideration of ethical guidelines. Make sure you review OpenAI's fine-tuning guide and comply with their usage policies.
Data Format
For fine-tuning the GPT-3 model, you need to format your training data as a series of messages in a conversation. Each message has a role
and content
field.
Here's an example of the required format for fine-tuning:
[
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": "How do I create a new document?"},
{"role": "assistant", "content": "To create a new document, click on the 'New Document' button."},
{"role": "user", "content": "Where can I find that button?"}
]
In this example, the conversation starts with a system message that sets the context for the assistant. It is followed by alternating user and assistant messages.
Make sure to include a variety of prompts and responses in your training data to help the model learn how to generate appropriate responses. You can include both the user and assistant messages during fine-tuning.
It's important to note that fine-tuning requires a large dataset, and OpenAI provides guidelines and recommendations for collecting and formatting training data. Make sure to refer to OpenAI's fine-tuning guide for detailed instructions on preparing your data.
Example 1: Asking for a recipe
[
{"role": "system", "content": "You are a recipe recommendation assistant."},
{"role": "user", "content": "Can you suggest a pasta recipe?"},
{"role": "assistant", "content": "Sure! How about trying a classic spaghetti carbonara?"},
{"role": "user", "content": "Great! What ingredients do I need?"},
{"role": "assistant", "content": "You'll need spaghetti, eggs, pancetta, Parmesan cheese, and black pepper."},
{"role": "user", "content": "Thanks! How do I cook it?"}
]
Example 2: Asking for travel recommendations
[
{"role": "system", "content": "You are a travel assistant."},
{"role": "user", "content": "I'm planning a trip to Paris. Any recommendations on places to visit?"},
{"role": "assistant", "content": "Absolutely! You must visit the Eiffel Tower, Louvre Museum, and Notre-Dame Cathedral."},
{"role": "user", "content": "Are there any good restaurants you can suggest?"},
{"role": "assistant", "content": "For a great dining experience, I recommend trying Le Jules Verne, L'Ambroisie, and Le Comptoir du Relais."},
{"role": "user", "content": "Thanks for the recommendations!"}
]
Example 3: Asking for programming help
[
{"role": "system", "content": "You are a programming assistant."},
{"role": "user", "content": "I'm having trouble with my Python code. Can you help me?"},
{"role": "assistant", "content": "Of course! Please share your code and let me know what issues you are facing."},
{"role": "user", "content": "Here's my code:\n\n```python\nprint('Hello, World!')\n```"},
{"role": "assistant", "content": "Your code looks fine. What error message are you getting, if any?"},
{"role": "user", "content": "I'm getting a 'SyntaxError: invalid syntax' error."}
]
Remember to structure your conversation data in a way that represents a back-and-forth interaction between the user and the assistant. The more diverse and representative your training data is, the better the fine-tuned model will be at generating relevant responses.
It's important to note that the maximum token limit for GPT-3.5 models is 4096 or 16k tokens. If your conversation exceeds this limit, you may need to truncate or omit some parts of the conversation to fit within the limit.